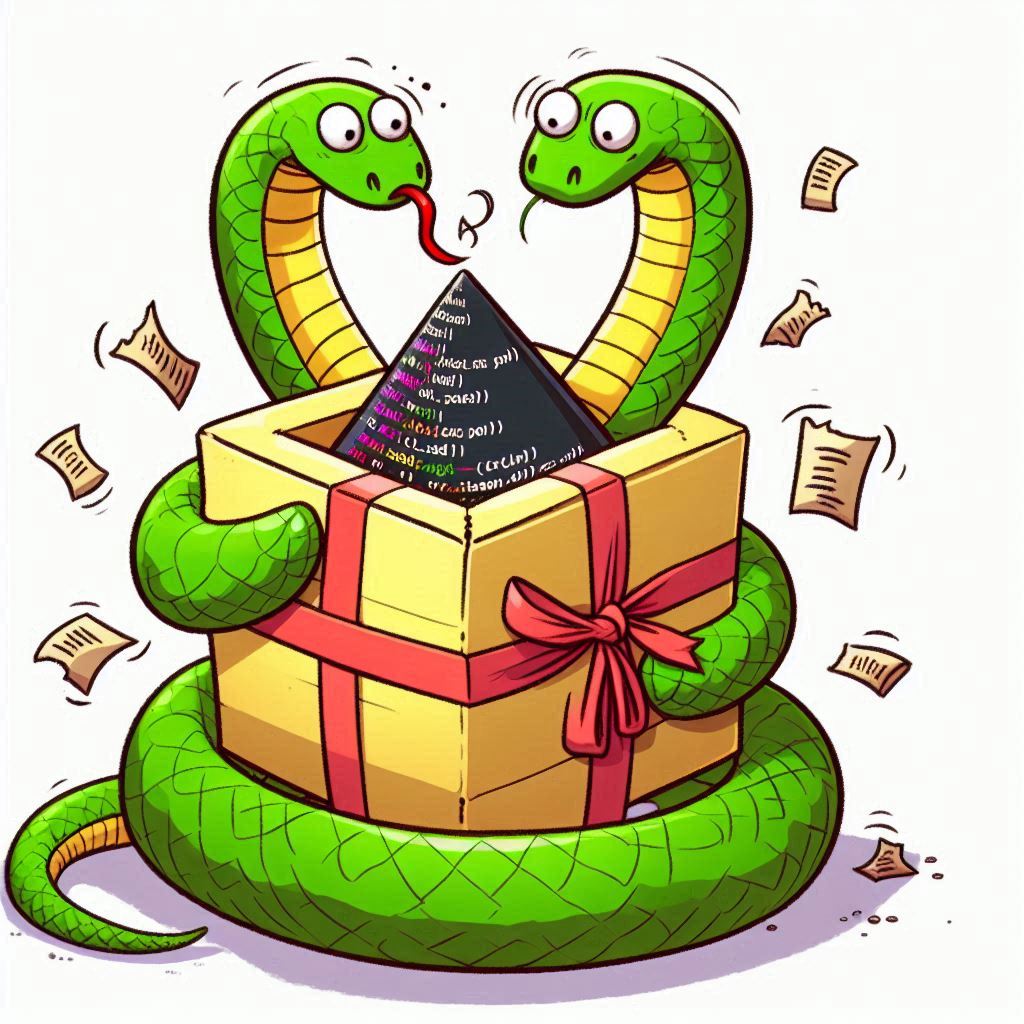
When unit testing, we usually mock interfaces to simplify our tests. However, there are cases where the dependency does not implement an interface, and you also do not have direct control over it. Sometimes you can get around it by mocking the actual class, but that’s not a reliable solution. Luckily for us, we can fix that by using wrappers.
What are wrappers?
Wrappers are classes that behave exactly as what it is wrapping. For example, say that you are working with the String class, your wrapper could look similarly to this:
In the constructor we receive an instance of the that we are wrapping, which we then store in a private field. Then we have the Substring method, which simply calls the original string method with the exact same parameters. And that’s all! We can do this for every other method or overload that we need from the String class.
How does a wrapper help me mocking?
If you are looking at the snippet above and thinking that’s just a bunch of unnecessary garbage: you are definitely right. It currently adds no value. So let’s come up with another situation. You are creating this new method that detects if a Type has the ToString method. Below you can see how that could be implemented.
Easy right? We simply return true or false if the method can be found or not. Now comes the tricky part: how would we write unit tests for it, which cover both cases (true and false)? For the scenario where it’s supposed to return true it’s quite easy, just use any type since they all implement ToString. However, it would be impossible for us to create tests to verify if it returns false when it doesn’t have said method. Of course, this is a very ridiculous case, but there are other situations where you would like to mock the dependency, such as to simplify your setup.
Gladly for us, we are now aware of wrappers, and can use them to easily test our new method. We just gotta add a new ingredient to our previous mix: an interface. Take a look:
With the use of the wrapper, we changed our method to receive an IType parameter. Since we are now using an interface, we can very easily mock it with any known mocking framework. The downside of wrappers is that depending on how many methods you need, they can get quite big. But still, that surely beats not having unit tests.